THE POKEDEX PROBLEM
Dated: 15/01/2023
Link to problem statement
Was watching this video, sipping on coffee and running some experiments in the background, when my brain said:
"I haven't done a technical interview in SO LONG! Have I fallen out of touch?"
Usually these thoughts come and go, but after a look at the problem it looked interesting because FINALLY I wasn't going to be inverting another binary tree.
Why not take a crack at it, afterall what does an engineer have to do on a sunday afternoon?
- The goal of this challenge is to:
- Have fun
- Understand where I stand in the Junior/Mid/Senior engineer categories
- HAVE FUN!
Reading the challenge, it has 3 parts and the first two look super easy (and I think I've written similar components a lot of times). A bit unsure how to do part 3 but I'll figure it out when I'm there.
Part one: Creating the row component
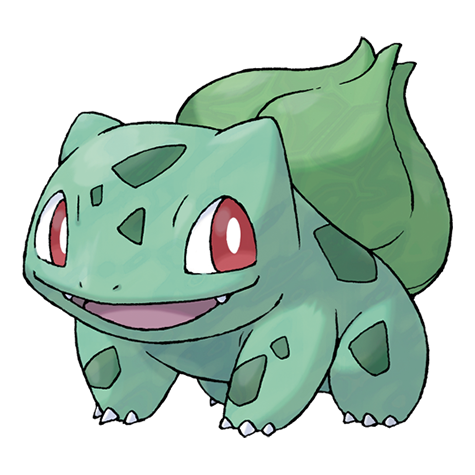
The image link provided in the problem description wasn't working, so I pulled another image that worked from the same website. Here's the code for the above:
//Using typeof bulbasaur because at this point in the challenge
//I haven't been told the exact type, maybe there could be additions,
//so not using an interface or a type declaration
const PokemonRow: React.FC<typeof bulbasaur> = ({ id, name, types, sprite }) => {
return (
<div className="flex flex-row p-4 bg justify-between bg-emerald-600 items-center font-bold">
<div>
{id}
</div>
<div>
{name.slice(0, 1).toUpperCase() + name.slice(1, name.length)}
</div>
<div>
{types.map(type => (
<div key={type}>
{type.slice(0, 1).toUpperCase() + type.slice(1, type.length) + ((types.indexOf(type) !== types.length-1) ? ',' : '')}
</div>
))}
</div>
<div className="w-12 h-auto">
<img
src={sprite}
alt={`Image of pokemon - ${name}`}
/>
</div>
</div>
)
}
Part two: Creating the table
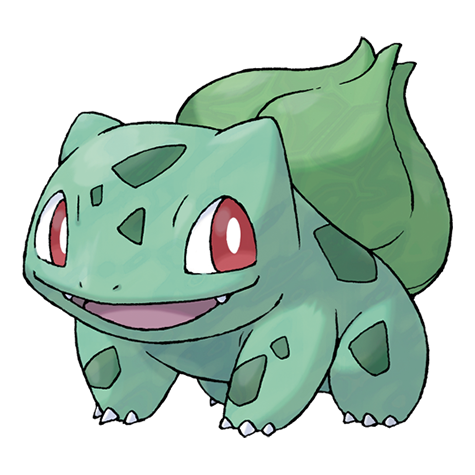
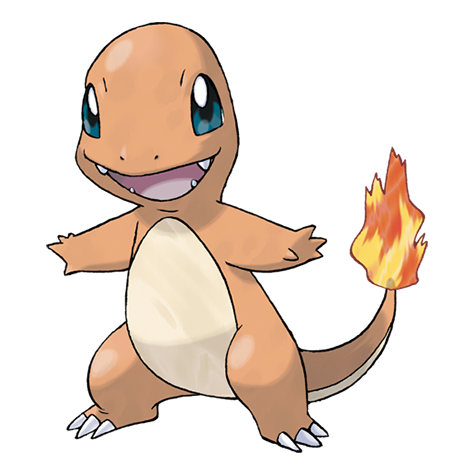
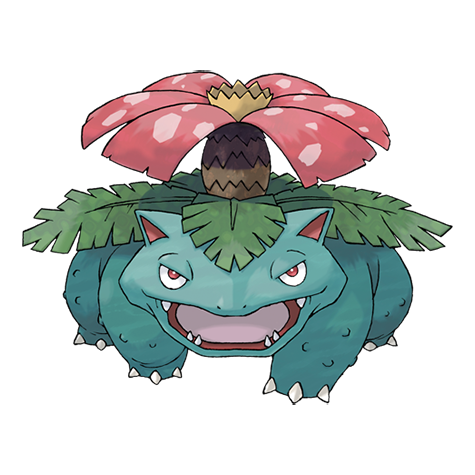
Since we know FOR SURE what the types are, we can add an interface here and use it for the array provided in part 2.
interface PokedexCharacter {
id: number,
name: string,
types: Array<string>,
sprite: string,
}
const pokemonArray: Array<PokedexCharacter> = [
{
id: 1,
name: "Bulbasaur",
types: ["grass"],
sprite: "https://assets.pokemon.com/assets/cms2/img/pokedex/full/001.png"
},
{
id: 2,
name: "Charmander",
types: ["fire"],
sprite: "https://assets.pokemon.com/assets/cms2/img/pokedex/full/004.png"
},
{
id: 3,
name: "Pidgeot",
types: ["normal", "flying"],
sprite: "https://assets.pokemon.com/assets/cms2/img/pokedex/full/018.png"
},
]
Part three: Creating a Selectable Component
The CSS above is not that good :P
const PokemonTypeSelection: React.FC<PokemonTypeSelectionProps> = (props) => {
const handleChange = (e: ChangeEvent<HTMLSelectElement>) => {
props.selectType(e.target.value);
}
return (
<select
className={`rounded-xl ${isDarkMode ? 'bg-gray-800' : 'bg-gray-300'} outline-none focus:outline-none w-32 p-2`}
value={props.selectedType}
onChange={handleChange}
>
{availableTypes.map(type => (
<option key={type} value={type}>
{type}
</option>
))}
</select>
)
}
const FilterablePokedexTable: React.FC<{ characters: Array<PokedexCharacter> }> = ({ characters }) => {
const [currentType, setCurrentType] = useState<string>();
const pokemonOfType = (pokemonType: string): Array<PokedexCharacter> => {
return characters.filter(char => char.types.includes(pokemonType));
}
return (
<>
<PokemonTypeSelection selectedType={currentType} selectType={setCurrentType} />
{currentType && <PokedexTable characters={pokemonOfType(currentType)} />}
</>
)
}
Thoughts
I thought part 3 would be more difficult but it turned out easy, hurray! :D
Doing this was fun, learned alternate ways to manage state when dealing with individual components. Good practice to write code this way IF you have a bit of time and good statefulness is important (for eg. this blogpost has HORRIBLE state vars - but works since the point is to publish it quickly)
Thanks for reading till here. I realize this was way more technical than first intended, and way more of checkpoint tracking than a blog, but it's a good starting point.